Justifying text in an Android app using a WebView instead of TextView
Justify text means aligning the text from the left and right hand sides, but the TextView of Android doesn't support text justification. If we want to use a TextView to display multiple lines, it look like as below: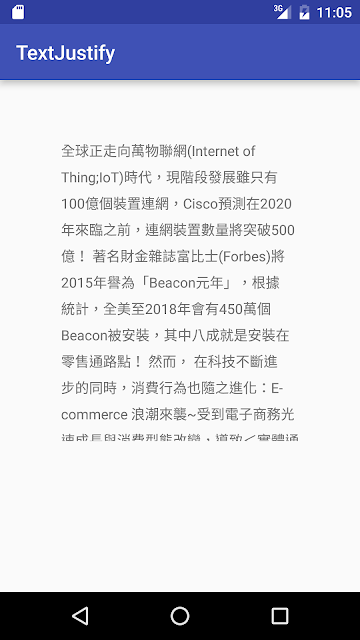
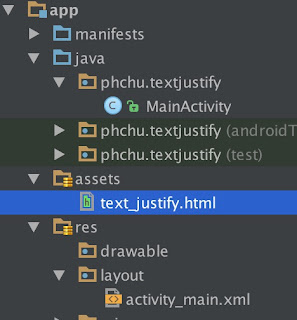
public class MainActivity extends AppCompatActivity { private static final String TEXT_JUSTIFY = "file:///android_asset/text_justify.html?info="; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); WebView desWebView = (WebView)findViewById(R.id.content_description_webview); String content = getResources().getString(R.string.content); desWebView.getSettings().setJavaScriptEnabled(true); desWebView.loadUrl(TEXT_JUSTIFY + content); } }
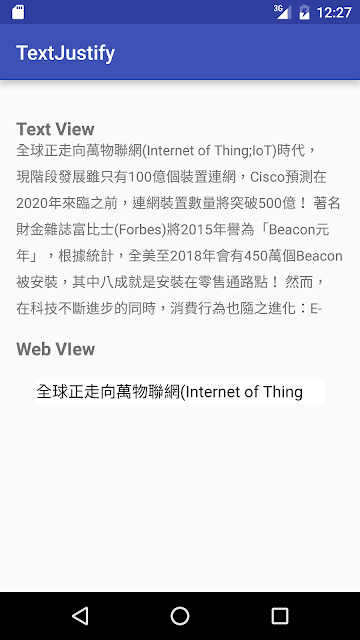
But there is also a problem: words after semicolon(";") of 1st line are disappear. The content string should be encoded before load in html page.
public class MainActivity extends AppCompatActivity { private static final String TEXT_JUSTIFY = "file:///android_asset/text_justify.html?info="; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); WebView desWebView = (WebView)findViewById(R.id.content_description_webview); String content = getResources().getString(R.string.content); desWebView.getSettings().setJavaScriptEnabled(true); try{ desWebView.loadUrl(TEXT_JUSTIFY + URLEncoder.encode(content, "utf-8")); }catch (UnsupportedEncodingException uee){ uee.printStackTrace(); } } }
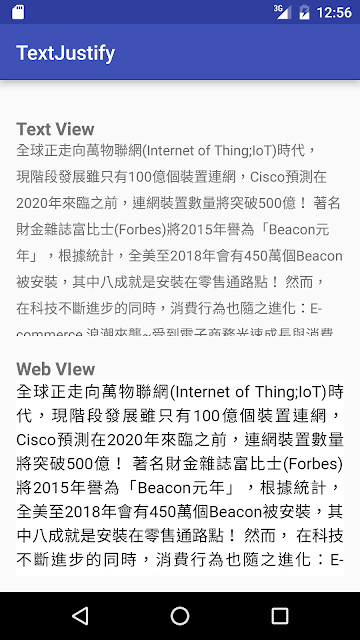
The method still have a problem, if the WebView on a layout with background color, it's not transparent.
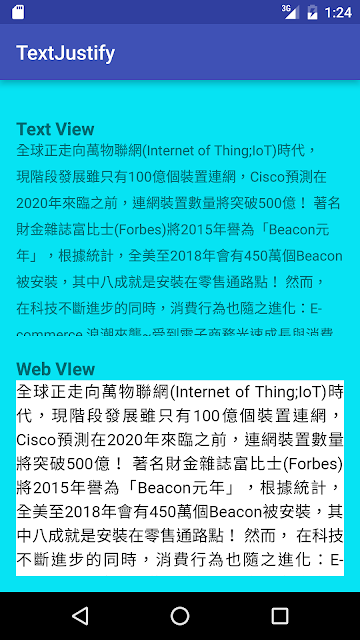
public class MainActivity extends AppCompatActivity { private static final String TEXT_JUSTIFY = "file:///android_asset/text_justify.html?info="; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); WebView desWebView = (WebView)findViewById(R.id.content_description_webview); String content = getResources().getString(R.string.content); desWebView.getSettings().setJavaScriptEnabled(true); desWebView.setBackgroundColor(0x00000000); try{ desWebView.loadUrl(TEXT_JUSTIFY + URLEncoder.encode(content, "utf-8")); }catch (UnsupportedEncodingException uee){ uee.printStackTrace(); } } }
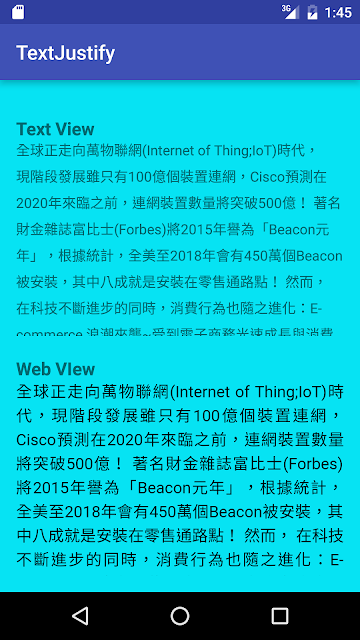
View Source Code on GitHub.
0 意見:
張貼留言