Building a Simple Video Player
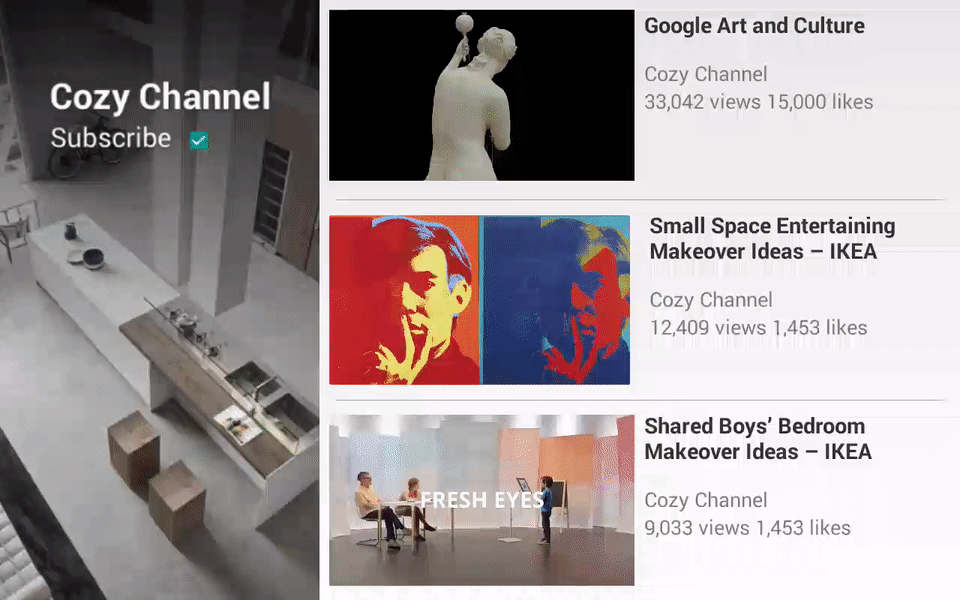
In this exercise,
- A small VideoView without media controller will auto play video at the beginning of launching app;
- If we single touch the VideoView, it will activate full screen mode and resume it at the stopped position in small VideoView;
- If we touch the back button in full screen mode, it will return to the small VideoView and and resume it at the stopped position in full screen mode.
Basic VideoView
- Create an empty project
- Create a folder named raw in res
- Add a local video in MP4 format in raw folder
- Create a MainActivity with layout(activity_main.xml)
activity_main.xml
MainActivity.java
public class MainActivity extends AppCompatActivity { private String TAG = "MainActivity"; private VideoView videoView; private String videoPath; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE); setContentView(R.layout.activity_main); videoView = (VideoView) this.findViewById(R.id.video_view); videoPath = "android.resource://" + getPackageName() + "/" + R.raw .google_arts_and_culture; videoView.setVideoURI(Uri.parse(videoPath)); videoView.start(); } }
Full Screen VideoView with MediaController
Create a FullscreenVideoActivity with layout(activity_fullscreen_video.xml)activity_fullscreen_video.xml
FullscreenVideoActivity.java
public class FullscreenVideoActivity extends Activity { private String TAG = "FullscreenVideoActivity"; private VideoView videoView; private MediaController mc; private String videoPath; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_fullscreen_video); mc = new MediaController(this); videoView = (VideoView) this.findViewById(R.id.fullscreen_video_view); videoView.setMediaController(mc); videoPath = "android.resource://" + getPackageName() + "/" + R.raw .google_arts_and_culture; videoView.setVideoURI(Uri.parse(videoPath)); videoView.start(); } }
From Basic VideoView into Full Screen Mode
Attaching a SimpleOnGestureListener to basic the VideoView for triggering full screen.MainActivity.java
public class MainActivity extends AppCompatActivity { private String TAG = "MainActivity"; private int stopPosition; private VideoView videoView; private String videoPath; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE); setContentView(R.layout.activity_main); videoView = (VideoView) this.findViewById(R.id.video_view); videoPath = "android.resource://" + getPackageName() + "/" + R.raw .google_arts_and_culture; videoView.setVideoURI(Uri.parse(videoPath)); videoView.start(); final GestureDetector gestureDetector = new GestureDetector(this, gestureListener); videoView.setOnTouchListener(new View.OnTouchListener() { @Override public boolean onTouch(View v, MotionEvent event) { return gestureDetector.onTouchEvent(event); } }); } final GestureDetector.SimpleOnGestureListener gestureListener = new GestureDetector.SimpleOnGestureListener() { @Override public boolean onDown(MotionEvent event) { return true; } @Override public boolean onSingleTapUp(MotionEvent event) { Log.e(TAG, "onSingleTapUp"); return true; } @Override public boolean onSingleTapConfirmed(MotionEvent e) { Log.e(TAG, "onSingleTapConfirmed"); videoView.pause(); stopPosition = videoView.getCurrentPosition(); Intent intent = new Intent(MainActivity.this, FullscreenVideoActivity.class); intent.putExtra("videoPath", videoPath); intent.putExtra("stopPosition", stopPosition); startActivity(intent); return true; } @Override public void onLongPress(MotionEvent e) { super.onLongPress(e); Log.e(TAG, "onLongPress"); } @Override public boolean onDoubleTap(MotionEvent e) { Log.e(TAG, "onDoubleTap"); return super.onDoubleTap(e); } }; }FullscreenVideoActivity.java
public class FullscreenVideoActivity extends Activity { private String TAG = "FullscreenVideoActivity"; private VideoView videoView; private MediaController mc; private String videoPath; private int stopPosition; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_fullscreen_video); Bundle params = getIntent().getExtras(); videoPath = params.getString("videoPath"); stopPosition = params.getInt("stopPosition"); mc = new MediaController(this); videoView = (VideoView) this.findViewById(R.id.fullscreen_video_view); videoView.setMediaController(mc); videoView.setVideoURI(Uri.parse(videoPath)); Log.e(TAG, "FullscreenVideoView intent stop position: " + stopPosition); videoView.seekTo(stopPosition); videoView.start(); } }
Back to Basic VideoView from Full Screen Mode
Overriding onResume() method of MainActivity for getting bundle with video stop position and file path from FullscreenVideoActivity.MainActivity.java
@Override protected void onResume() { super.onResume(); Bundle params = getIntent().getExtras(); if(null != params){ Log.e(TAG, "VideoView intent stop position: " + stopPosition); videoPath = params.getString("videoPath"); stopPosition = params.getInt("stopPosition"); videoView.seekTo(stopPosition); videoView.start(); } }FullscreenVideoActivity.java
Overriding onBackPressed() method of FullscreenVideoActivity for going back to MainActivity with video stop position and file path.
@Override public void onBackPressed() { super.onBackPressed(); videoView.pause(); stopPosition = videoView.getCurrentPosition(); Intent intent = new Intent(FullscreenVideoActivity.this, MainActivity.class); intent.putExtra("videoPath", videoPath); intent.putExtra("stopPosition", stopPosition); startActivity(intent); }
Result
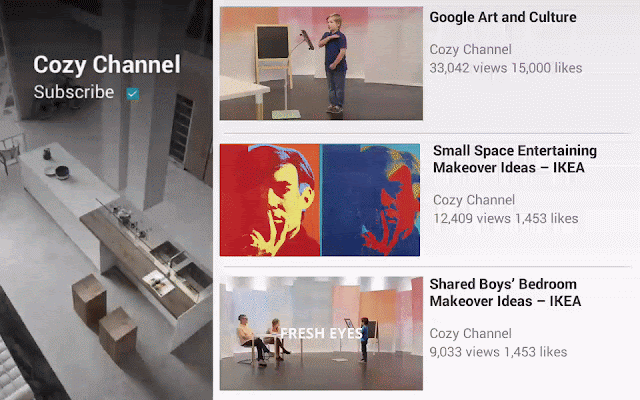
View on GitHub
0 意見:
張貼留言